-
-
Notifications
You must be signed in to change notification settings - Fork 28
Country Picker Dialog
Harsh B. Bhakta edited this page Aug 6, 2020
·
6 revisions
For some reason, if you don't want to use default Country Picker View and want to launch dialog on your own. You can use extension function to launch the dialog.
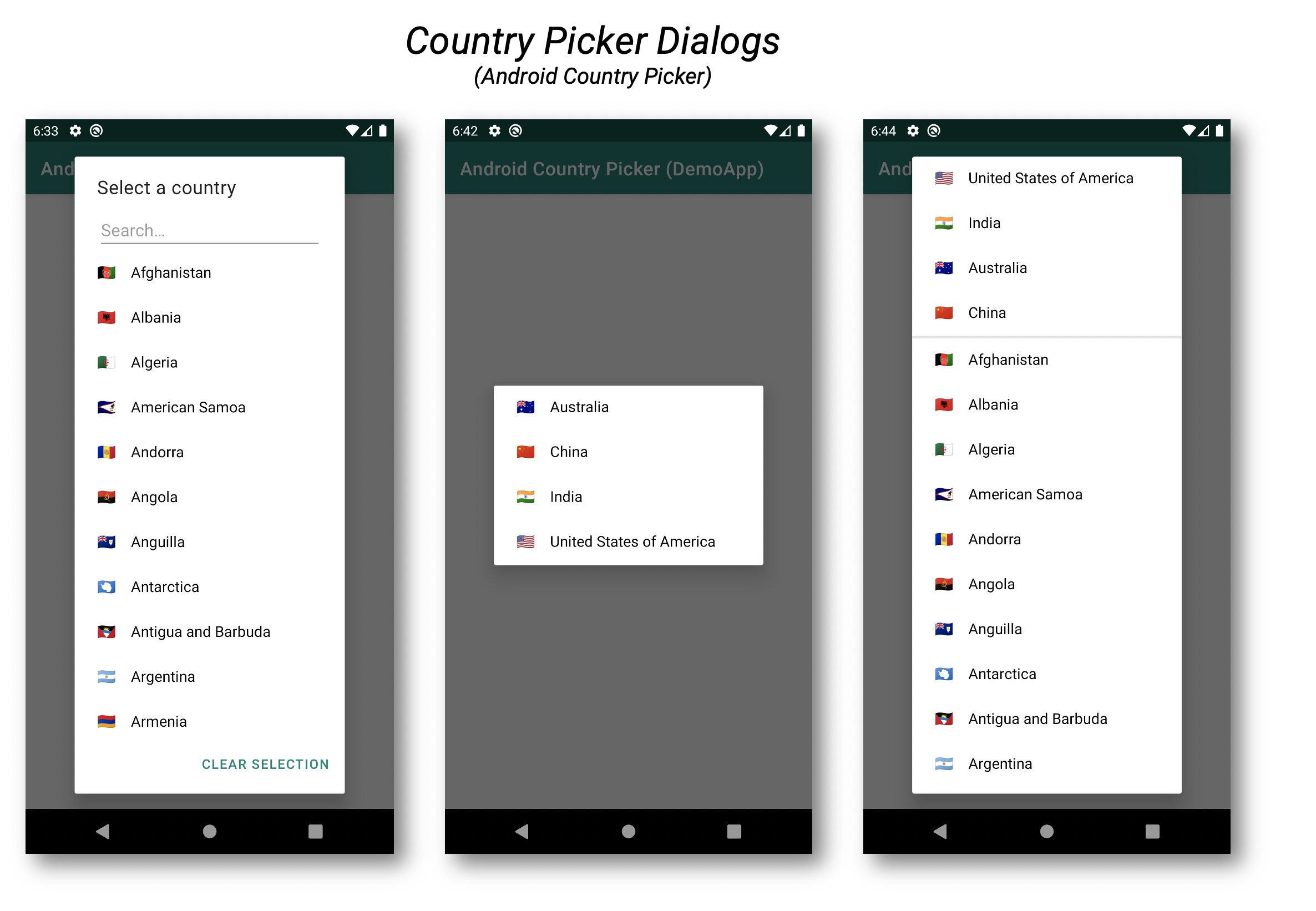
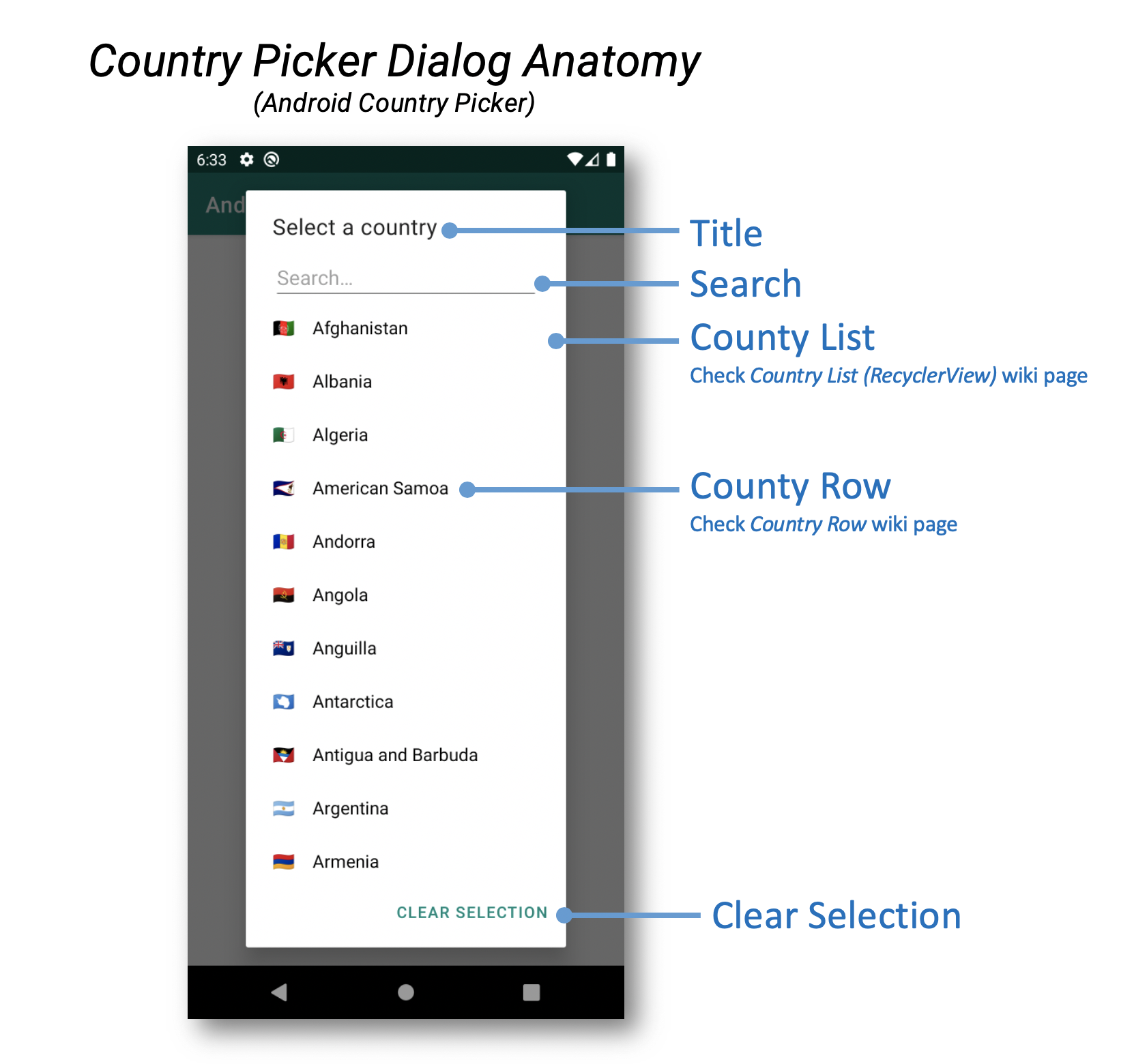
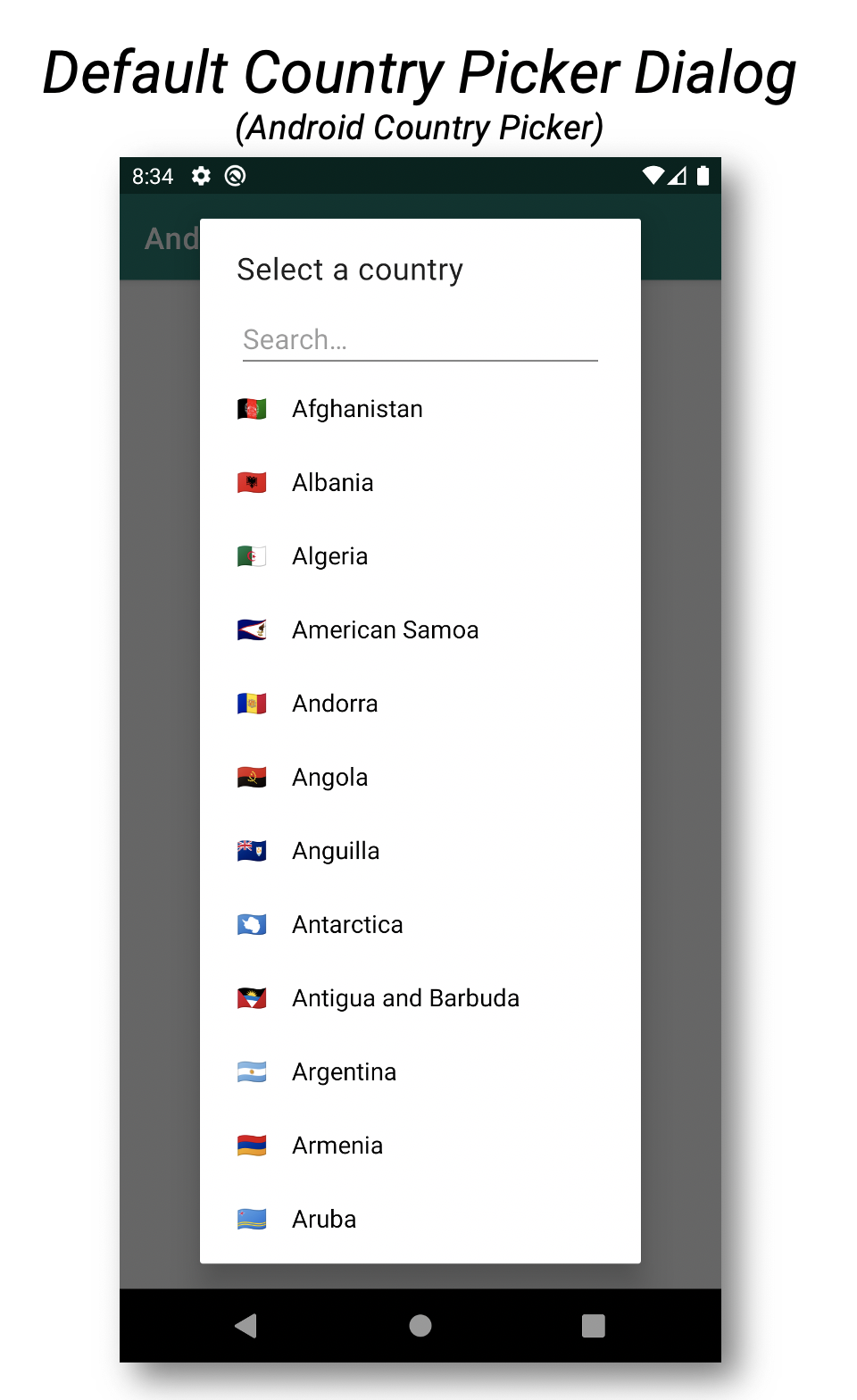
-
Launch using Extension function (source)
With Kotlin in action, you can launch dialog using,
context.launchCountryPickerDialog { selectedCountry: CPCountry? ->
// your code to handle selected country
}
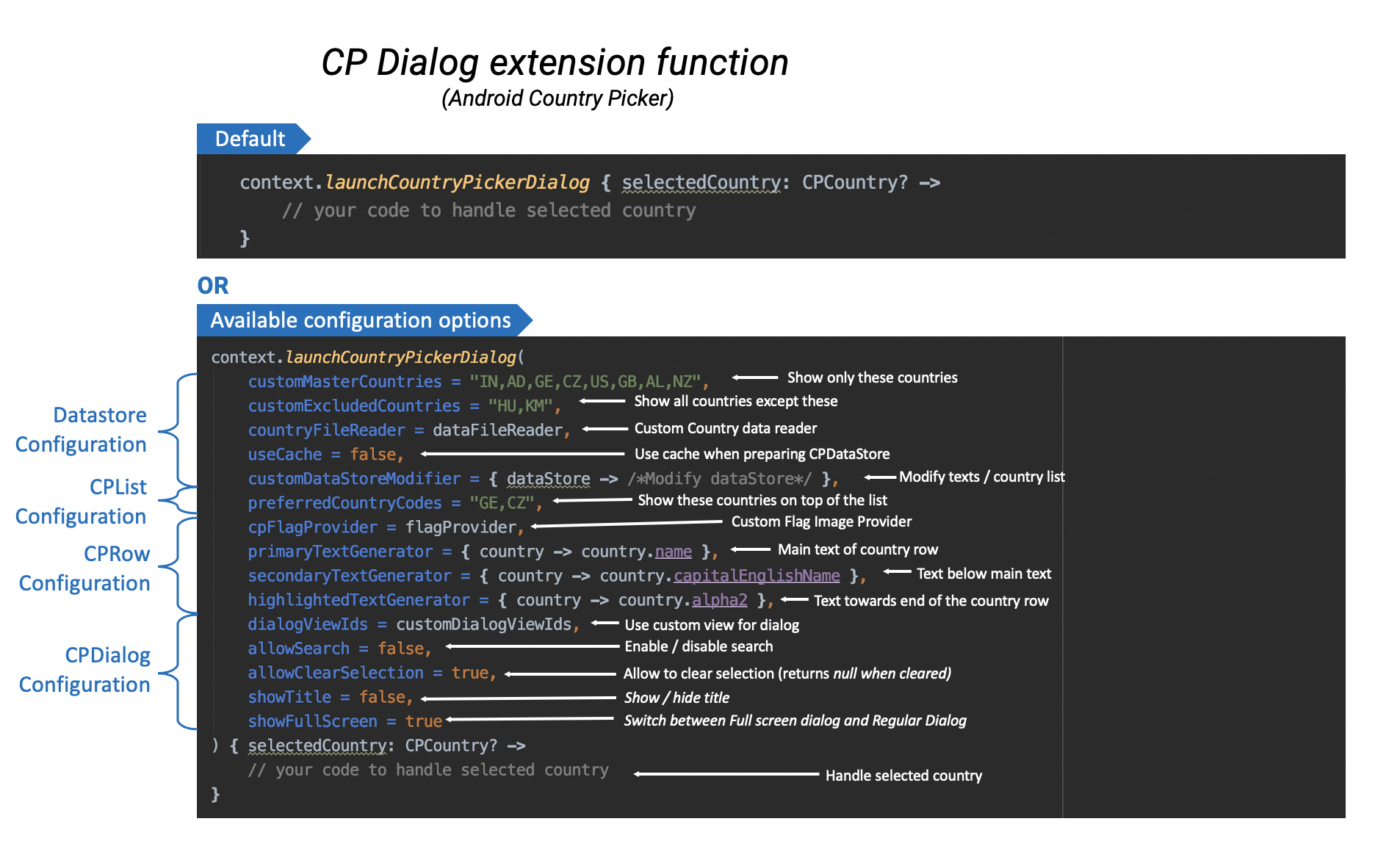
- Read more about [Data Store Configuration](todo: add link)
- Read more about CP List Configuration
- Read more about CP Row Configuration
- Read more about [CP Dialog Configuration](todo: add link) - below
Configuration - CPDialogConfig
source
- This can be used to apply your custom dialog layout when dialog is launched.
- By default, it uses default
cp_dialog.xml
and it's children views - To use your custom layout do following
- Create a layout file (e.g.
custom_cp_dialog.xml
) - Add following view(s) with custom IDs - these view IDs will be used later
- RecyclerView
- TextView for dialog title (optional)
- EditText for filter query (optional)
- ImageView to clear edit text (optional)
- Button to clear selection (optional)
- Create instance of
CPDialogViewIds
using these view IDs-
val cpDialogViewIds = CPDialogViewIds( R.layout.id_of_layout_file, R.id.id_of_recyclerView, R.id.id_of_title_textview, // optional R.id.id_of_filter_editText, // optional R.id.id_of_clearQuery_imageView, // optional R.id.id_of_clearSelection_button // optional )
-
- Provide this
cpDialogViewIds
through extension functions [todo: add link] orCPDialogHelper
[todo: add link].
- Create a layout file (e.g.
- Enable / disable search feature
- By default, search is
enabled
- Options
-
allowSearch = true
to enable search -
allowSearch = false
to disable search
-
- Provide this through extension functions [todo: add link] or
CPDialogHelper
[todo: add link].
- Show / hide
Clear Selection
button - By default,
showTitle = false
- When user click on
Clear Selection
button, callback will be triggered withselectedCountry = null
- Options
-
allowClearSelection = true
to showClear Selection
button -
allowClearSelection = false
to hideClear Selection
button
-
- Provide this through extension functions [todo: add link] or
CPDialogHelper
[todo: add link].
- Show / hide dialog
title
- By default,
showTitle = true
- Options
-
showTitle = true
to show dialogtitle
-
showTitle = false
to hide dialogtitle
-
- Provide this through extension functions [todo: add link] or
CPDialogHelper
[todo: add link].
- Show regular size / full size dialog
- By default,
showFullScreen = false
- Options
-
showFullScreen = true
to show full screen dialog -
showFullScreen = false
to show regular size dialog
-
- Provide this through extension functions [todo: add link] or
CPDialogHelper
[todo: add link]. - Example:
-
- Notes
- Do not use fullScreen if you have small master country list that do not fill entire screen.
Launch using CPDialogHelper
(source)
To launch dialog using Extension function (todo: add link) keeps your code clean. If you want more granular control over instance creation, then you can do following:
// Check CPDataStoreGenerator for available configuration
val cpDataStore = CPDataStoreGenerator.generate(context)
// Check CPListConfig for available configuration
val cpListConfig = CPListConfig()
// Check CPRowConfig for available configuration
val cpRowConfig: CPRowConfig = CPRowConfig()
// Check CPDialogConfig for available configuration
val cpDialogConfig: CPDialogConfig = CPDialogConfig()
// Prepare dialogHelper
val cpDialogHelper = CPDialogHelper(
cpDataStore = cpDataStore, // required
cpListConfig = cpListConfig, // Default: CPListConfig()
cpRowConfig = cpRowConfig, // Default: CPRowConfig()
cpDialogConfig = cpDialogConfig // Default: CPDialogConfig()
){ selectedCountry: CPCountry? ->
// required: handle selected country
// note: this is nullable to support clear selection
}
// prepare dialog instance
val dialog = cpDialogHelper.createDialog(context)
// show dialog
dialog.show()